Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
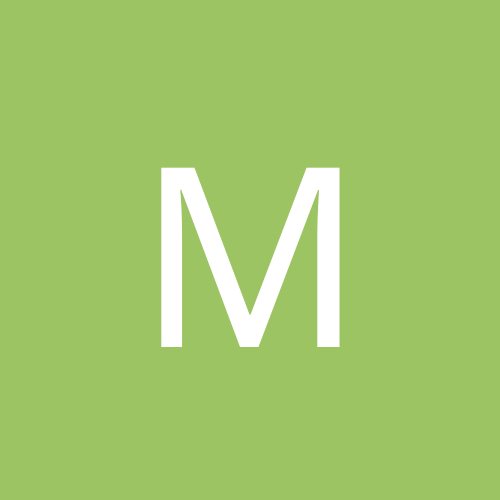
Md5
Por
marcio.theis, em Delphi
Este tópico foi arquivado e está fechado para novas respostas.
Por
marcio.theis, em Delphi
Ao usar o fórum, você concorda com nossos Termos e condições.