Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
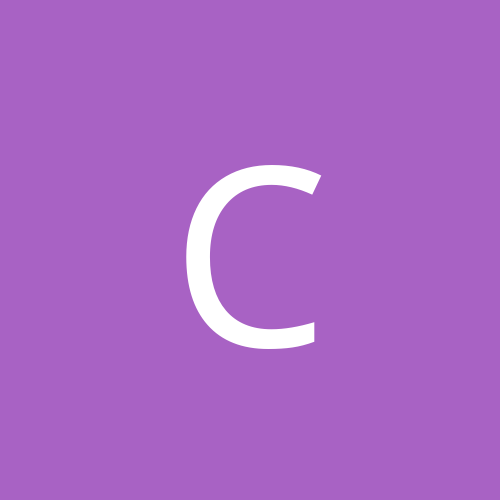
Form em Div Carregado por Ajax
Por
Cleberson Ramos, em Javascript
Este tópico foi arquivado e está fechado para novas respostas.
Por
Cleberson Ramos, em Javascript
Ao usar o fórum, você concorda com nossos Termos e condições.