Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
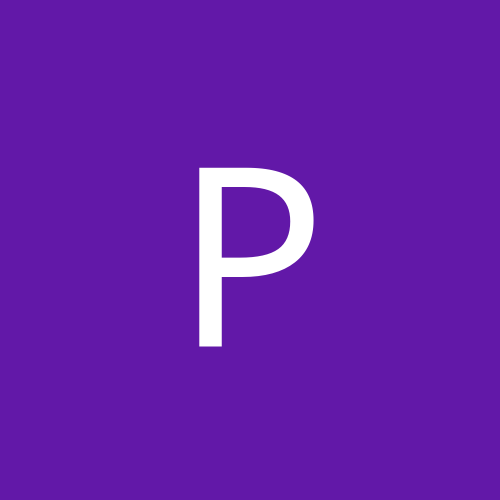
Exibir imagens dinâmicas e uma página HTML
Por
Prove Yourself, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
Prove Yourself, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.