Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
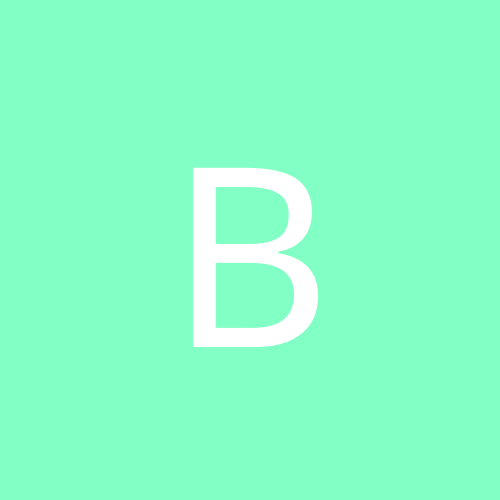
Envio de Arquivo XML
Por
Bruno - E-Storage - Google, em .NET
Este tópico foi arquivado e está fechado para novas respostas.
Por
Bruno - E-Storage - Google, em .NET
Ao usar o fórum, você concorda com nossos Termos e condições.