Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
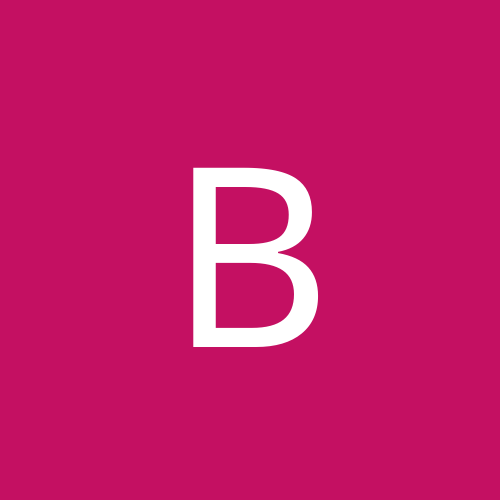
crop imagem
Por
BrunoVieira, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
BrunoVieira, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.