Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
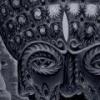
[Resolvido] [Código] sprintf com std::strings
Por
VictorCacciari, em C/C++
Este tópico foi arquivado e está fechado para novas respostas.
Por
VictorCacciari, em C/C++
Ao usar o fórum, você concorda com nossos Termos e condições.