Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
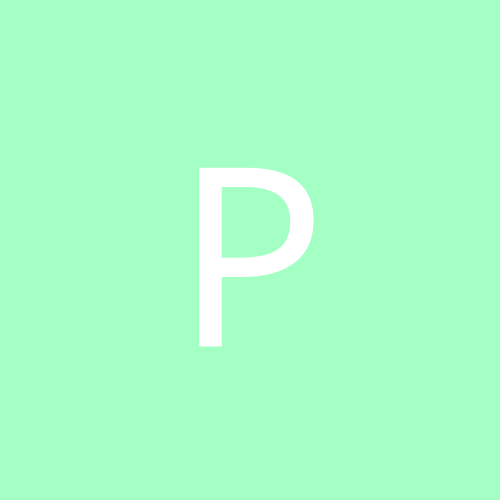
Function Facebook
Por
Patrique, em ASP Clássico
Este tópico foi arquivado e está fechado para novas respostas.
Por
Patrique, em ASP Clássico
Ao usar o fórum, você concorda com nossos Termos e condições.