Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
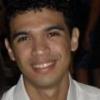
Inscrição em três passos
Por
vieira.rrafael, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
vieira.rrafael, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.