Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
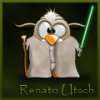
[Resolvido] [Código] Converter números para texto em C+
Por
Renato Utsch, em C/C++
Este tópico foi arquivado e está fechado para novas respostas.
Por
Renato Utsch, em C/C++
Ao usar o fórum, você concorda com nossos Termos e condições.