Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
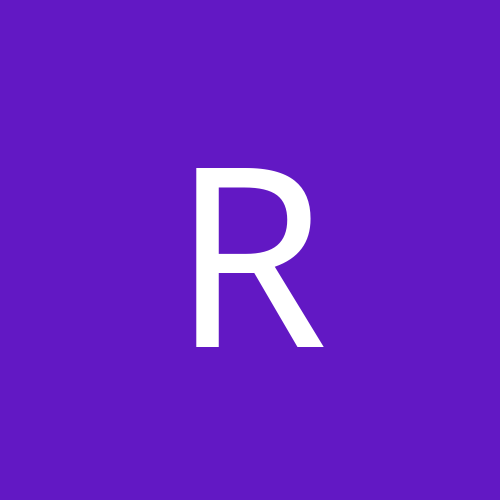
[Resolvido] Windows Phone 7: como se comunicar com um aplicativo deskt
Por
RSS iMasters, em .NET
Este tópico foi arquivado e está fechado para novas respostas.
Por
RSS iMasters, em .NET
Ao usar o fórum, você concorda com nossos Termos e condições.