Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
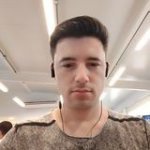
Classe para Validação de CPF
Por
Jonatã Cioni, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
Jonatã Cioni, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.