Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
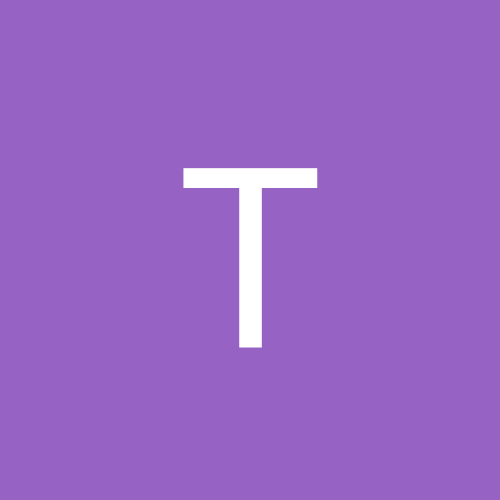
Classe Upload
Por
Thiago Retondar, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
Thiago Retondar, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.