Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
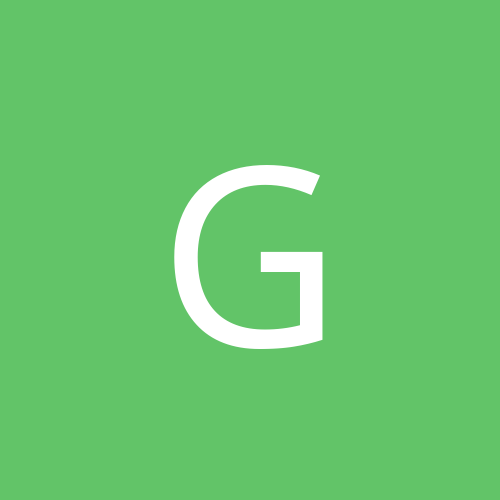
[Resolvido] Crud - PHP OO + PDO
Por
Guilherme_90, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
Guilherme_90, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.