Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
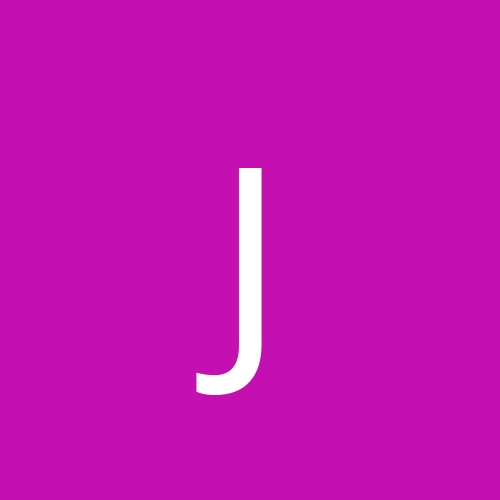
compilar
Por
Jonathan Daniel, em C/C++
Este tópico foi arquivado e está fechado para novas respostas.
Por
Jonathan Daniel, em C/C++
Ao usar o fórum, você concorda com nossos Termos e condições.