Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
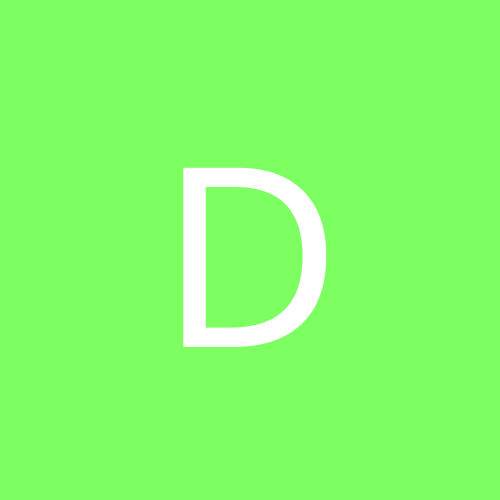
Problemas com Algoritmo de Ordenação por Inserção Binária
Por
Diego Koscky, em C/C++
Este tópico foi arquivado e está fechado para novas respostas.
Por
Diego Koscky, em C/C++
Ao usar o fórum, você concorda com nossos Termos e condições.