Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
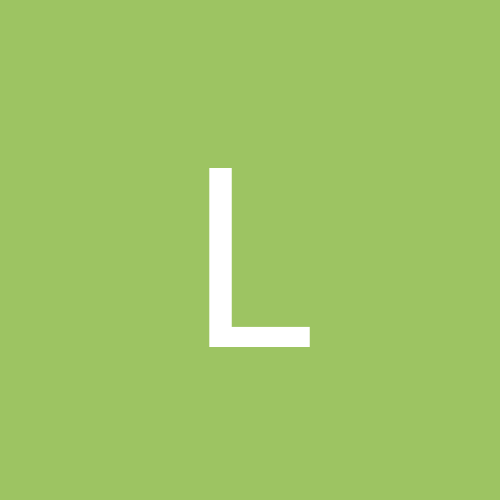
Carrinho de compras, opções tamanho e tipo.
Por
Luiz Eduardo_8047, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
Luiz Eduardo_8047, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.