Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
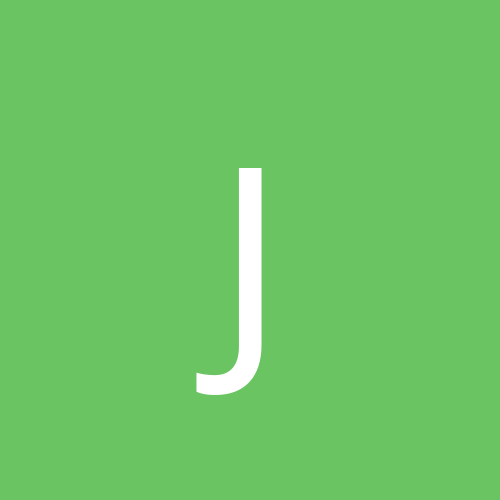
Delegates e eventos entre Form()
Por
jxfdasilva, em .NET
Este tópico foi arquivado e está fechado para novas respostas.
Por
jxfdasilva, em .NET
Ao usar o fórum, você concorda com nossos Termos e condições.