Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
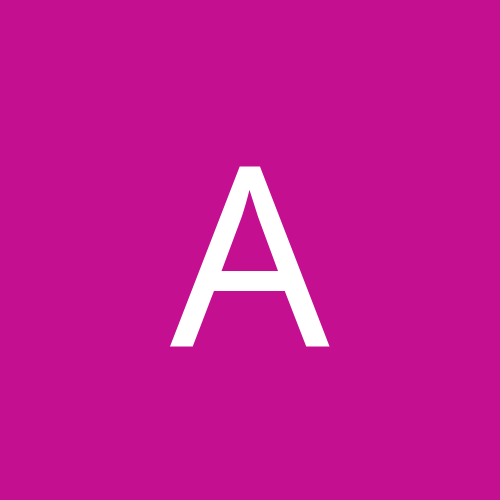
Analises de algoritmos de ordenações
Por
arsenium123, em C/C++
Este tópico foi arquivado e está fechado para novas respostas.
Por
arsenium123, em C/C++
Ao usar o fórum, você concorda com nossos Termos e condições.