Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
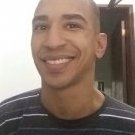
Preciso acessar com javascript a função que abre a janela de seleção d
Por
DinhoPHP, em Javascript
Recommended Posts
-
Conteúdo Similar
-
Por violin101
Caros amigos, saudações.
Estou com uma dúvida, referente cálculo de valores em tempo real.
Tenho uma rotina, que faz o cálculo, o problema é mostrar o resultado.
Quero mostrar o RESULTADO assim: 0,00 ou 0.00
Abaixo posto o código.
jQuery('input').on('keyup',function(){ //Remover ponto e trocar a virgula por ponto var m = document.getElementById("pgRest").value; while (m.indexOf(".") >= 0) { m = m.replace(".", ""); } m = m.replace(",","."); //Remover ponto e trocar a virgula por ponto var j = document.getElementById("pgDsct").value; while (j.indexOf(".") >= 0) { j = j.replace(".", ""); } j = j.replace(",","."); m = parseFloat(jQuery('#pgRest').val() != '' ? jQuery('#pgRest').val() : 0); j = parseFloat(jQuery('#pgDsct').val() != '' ? jQuery('#pgDsct').val() : 0); //Mostra o Resultado em Tempo Real jQuery('#pgTroco').val(m - j); <<=== aqui estou errando })
Grato,
Cesar
-
Por violin101
Caro amigos, saudações.
Tenho uma tabela escrita em JS que funciona corretamente.
Minha dúvida:
- como devo fazer para quando a Tabela HTML estiver vazia, exibir o LOGO da Empresa ?
Abaixo posto o script:
document.addEventListener( 'keydown', evt => { if (!evt.ctrlKey || evt.key !== 'i' ) return;// Não é Ctrl+A, portanto interrompemos o script evt.preventDefault(); //Chama a Função Calcular Qtde X Valor Venda calcvda(); var idProdutos = document.getElementById("idProdutos").value; var descricao = document.getElementById("descricao").value; var prd_unid = document.getElementById("prd_unid").value; var estoque_atual = document.getElementById("estoque_atual").value; var qtde = document.getElementById("qtde").value; var vlrunit = document.getElementById("vlrunit").value; var vlrtotals = document.getElementById("vlrtotal").value; var vlrtotal = vlrtotals.toLocaleString('pt-br', {minimumFractionDigits: 2}); if(validarConsumo(estoque_atual)){ //Chama a Modal com Alerta. $("#modal_qtdemaior").modal(); } else { if(qtde == "" || vlrunit == "" || vlrtotal == ""){ //Chama a Modal com Alerta. $("#modal_quantidade").modal(); } else { //Monta a Tabela com os Itens html = "<tr style='font-size:13px;'>"; html += "<td width='10%' height='10' style='text-align:center;'>"+ "<input type='hidden' name='id_prds[]' value='"+idProdutos+"'>"+idProdutos+"</td>"; html += "<td width='47%' height='10'>"+ "<input type='hidden' name='descricao[]' value='"+descricao+"'>"+descricao+ "<input type='hidden' name='esp[]' value='"+prd_unid+"'> - ESP:"+prd_unid+ "<input type='hidden' name='estoq[]' value='"+estoque_atual+"'></td>"; html += "<td width='10%' height='10' style='text-align:center;'>"+ "<input type='hidden' name='qtde[]' value='"+qtde+"'>"+qtde+"</td>"; html += "<td width='12%' height='10' style='text-align:right;'>"+ "<input type='hidden' name='vlrunit[]' value='"+vlrunit+"'>"+vlrunit+"</td>"; html += "<td width='14%' height='10' style='text-align:right;'>"+ "<input type='hidden' name='vlrtotal[]' value='"+vlrtotal+"'>"+vlrtotal+"</td>"; html += "<td width='12%' height='10' style='text-align:center;'>"+ "<button type='button' class='btn btn-uvas btn-remove-produto' style='margin-right:1%; padding:1px 3px; font-size:12px;' title='Remover Item da Lista'>"+ "<span class='fa fa-minus' style='font-size:12px;'></span></button></td>"; html += "</tr>"; $("#tbventas tbody").append(html); //Função para Somar os Itens do Lançamento somar(); $("#idProdutos").val(null); $("#descricao").val(null); $("#prd_unid").val(null); $("#qtde").val(null); $("#vlrunit").val(null); $("#vlrtotal").val(null); $("#idProdutos").focus(); //Se INCLUIR NOVO produto - Limpa a Forma de Pagamento $("#pgSoma").val(null); $("#pgRest").val(null); $("#pgDsct").val(null); $("#pgTroco").val(null); $("#tbpagar tbody").empty(); }//Fim do IF-qtde }//Fim do Validar Consumo });//Fim da Função btn-agregar
Grato,
Cesar
-
Por violin101
Caros amigos, saudações.
Estou com uma pequena dúvida se é possível ser realizado.
Preciso passar 2 IDs para o Sistema executar a função, estou utilizando desta forma e gostaria de saber como faço via JS para passar os parâmetro que preciso.
Observação:
Dentro da TABELA utilizei 2 Forms, para passar os IDS que preciso, funcionou conforme código abaixo.
<div class="card-body"> <table id="tab_clie" class="table table-bordered table-hover"> <thead> <tr> <th style="text-align:center; width:10%;">Pedido Nº</th> <th style="text-align:center; width:10%;">Data Pedido</th> <th style="text-align:center; width:32%;">Fornecedor</th> <th style="text-align:center; width:10%;">Status</th> <th style="text-align:center; width:5%;">Ação</th> </tr> </thead> <tbody> <?php foreach ($results as $r) { $dta_ped = date(('d/m/Y'), strtotime($r->dataPedido)); switch ($r->pd_status) { case '1': $status = ' Aberto '; $txt = '#FFFFFF'; //Cor: Branco $cor = '#000000'; //Cor: Preta break; case '2': $status = 'Atendido Total'; $txt = '#FFFFFF'; //Cor: Branco $cor = '#086108'; //Cor: Verde break; case '3': $status = 'Atendido Parcial'; $txt = '#000000'; //Cor: Branco $cor = '#FEA118'; //Cor: Amarelo break; default: $status = 'Cancelado'; $txt = '#FFFFFF'; //Cor: Branco $cor = '#D20101'; //Cor: Vermelho break; } echo '<tr>'; echo '<td width="10%" height="10" style="text-align:center;">'.$r->pd_numero.'</td>'; echo '<td width="10%" height="10" style="text-align:center;">'.$dta_ped.'</td>'; echo '<td width="32%" height="10" style="text-align:left;">'.$r->nome.'</td>'; echo '<td width="10%" height="10" style="text-align:left;"><span class="badge" style="color:'.$txt.'; background-color:'.$cor.'; border-color:'.$cor.'">'.$status.'</span></td>'; echo '<td width="5%" style="text-align:center;">'; ?> <div class="row"> <?php if($this->permission->checkPermission($this->session->userdata('permissao'), 'vPedido')){ ?> <form action="<?= base_url() ?>compras/pedidos/visualizar" method="POST" > <input type="hidden" name="idPedido" value="<?php echo $r->idPedidos; ?>"> <input type="hidden" name="nrPedido" value="<?php echo $r->pd_numero; ?>"> <button class="btn btn-warning" title="Visualizar" style="margin-left:50%; padding: 1px 3px;"><i class="fa fa-search icon-white"></i></button> </form> <?php } if($this->permission->checkPermission($this->session->userdata('permissao'), 'ePedido')){ ?> <form action="<?= base_url() ?>compras/pedidos/editar" method="POST" > <input type="hidden" name="idPedido" value="<?php echo $r->idPedidos; ?>"> <input type="hidden" name="nrPedido" value="<?php echo $r->pd_numero; ?>"> <button class="btn btn-primary" title="Editar" style="margin-left:50%; padding: 1px 3px;"><i class="fa fa-edit icon-white"></i></button> </form> <?php } ?> </div> <?php echo '</td>'; echo '</tr>'; } ?> </tbody> </table> </div>
Grato,
Cesar.
-
Por belann
Olá!
Estou usando o editor quill em uma página html, sem fazer a instalação com npm, mas usando as api´s via internet com http, no entanto não consigo fazer a tecla enter funcionar para mudança de linha, tentei essa configuração abaixo, mas não funcionou.
modules: { syntax: true, toolbar: '#toolbar-container', keyboard: { bindings: { enter: { key: 13, handler: function(range, context) { quill.formatLine(range.index, range.length, { 'align': '' }); } }
-
Por violin101
Caros amigos, saudações.
Gostaria de poder tirar uma dúvida com os amigos.
Como faço uma função para Comparar a Data Digitada pelo o Usuário com a Data Atual ?
Data Digitada: 01/09/2024
Exemplo:
25/09/2024 é menor que DATA Atual ====> mensagem: informe uma data válida.
25/09/2024 é igual DATA Atual ===> o sistema libera os INPUT's.
Como faço uma comparação com a Data Atual, para não Deixar Gravar Data retroativa a data Atual.
Grato,
Cesar
-