Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
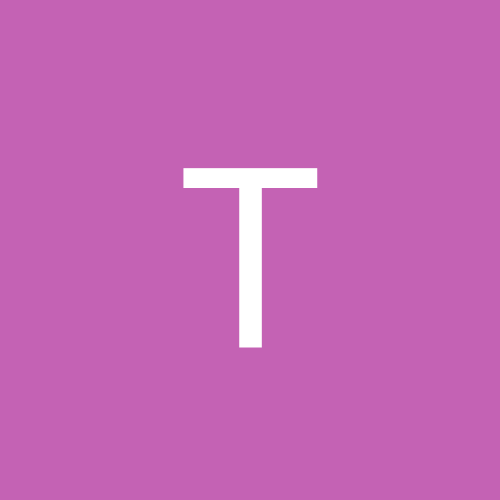
Backup automatico para Google Drive com PHP
Por
Thiago Lenzi, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
Thiago Lenzi, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.