Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
- 0
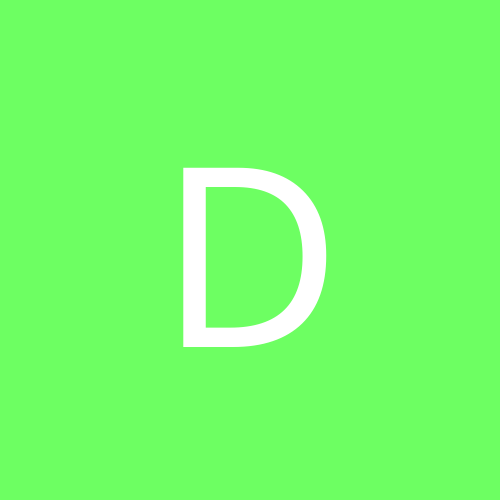
Modelar uma instante de tempo definida pela hora, minuto e segundo.
Perguntado por
Davide Silva
Este tópico foi arquivado e está fechado para novas respostas.
Perguntado por
Davide Silva
Ao usar o fórum, você concorda com nossos Termos e condições.
Oi galera, o código em baixo funciona direitinho, com pre-inicialização. O problema que eu estou tendo é como faço para implementar
● método que imprime a instancia em formato de 12 horas (AM/ PM)
● previousSecond()
● nextMinute()
● previousMinute()
● nextHour()
● previousHour()
/* Test Driver for the Time class */
#include <iostream>
#include "Time.h" // include header of Time class
using namespace std;
int main() {
Time t_2;
int minut;
int seg;
int hor;
cout<< "\n Informe a hora: ";
cin>>hor;
t_2.setHour(hor);
cout<< "\n Informe o minuto: ";
cin>>minut;
t_2.setMinute(minut);
cout<< "\n Informe o segundo: ";
cin>>seg;
t_2.setSecond(seg);
cout<<"Object t1 state: \n";
cout << "Hour is " << t_2.getHour() << endl;
cout << "Minute is " << t_2.getMinute() << endl;
cout << "Second is " << t_2.getSecond() << endl;
return 0;
}
//=======================================================================================
/* Header for the Time class (Time.h) */
#ifndef TIME_H // Include this "block" only if TIME_H is NOT defined
#define TIME_H // Upon the first inclusion, define TIME_H so that
// this header will not get included more than once
class Time {
private: // private section
// private data members
int hour; // 0 - 23
int minute; // 0 - 59
int second; // 0 - 59
public: // public section
// public member function prototypes
//Time(int h = 0, int m = 0, int s = 0); // Constructor with default values
int getHour() const; // public getter for private data member hour
void setHour(int h); // public setter for private data member hour
int getMinute() const; // public getter for private data member minute
void setMinute(int m); // public setter for private data member minute
int getSecond() const; // public getter for private data member second
void setSecond(int s); // public setter for private data member second
void setTime(int h, int m, int s); // set hour, minute and second
void print() const; // Print a description of this instance in "hh:mm:ss"
void nextSecond(); // Increase this instance by one second
}; // need to terminate the class declaration with a semicolon
#endif // end of "#ifndef" block
//======================================================================================
/* Implementation for the Time Class (Time.cpp) */
#include <iostream>
#include <iomanip>
#include "Time.h" // include header of Time class
using namespace std;
// Constructor with default values. No input validation
/*Time::Time(int h, int m, int s) {
hour = h;
minute = m;
second = s;
}
*/
// public getter for private data member hour
int Time::getHour() const {
return hour;
}
// public setter for private data member hour. No input validation
void Time::setHour(int h) {
hour = h;
}
// public getter for private data member minute
int Time::getMinute() const {
return minute;
}
// public setter for private data member minute. No input validation
void Time::setMinute(int m) {
minute = m;
}
// public getter for private data member second
int Time::getSecond() const {
return second;
}
// public setter for private data member second. No input validation
void Time::setSecond(int s) {
second = s;
}
// Set hour, minute and second. No input validation
void Time::setTime(int h, int m, int s) {
hour = h;
minute = m;
second = s;
}
// Print this Time instance in the format of "hh:mm:ss", zero filled
void Time::print() const {
cout << setfill('0'); // zero-filled, need <iomanip>, sticky
cout << setw(2) << hour // set width to 2 spaces, need <iomanip>, non-sticky
<< ":" << setw(2) << minute
<< ":" << setw(2) << second << endl;
}
// Increase this instance by one second
void Time::nextSecond() {
++second;
if (second >= 60) {
second = 0;
++minute;
}
if (minute >= 60) {
minute = 0;
++hour;
}
if (hour >= 24) {
hour = 0;
}
}
Desde já obrigado
Compartilhar este post
Link para o post
Compartilhar em outros sites