Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
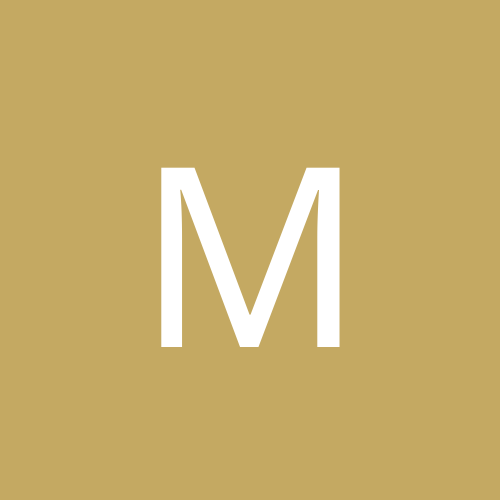
[Código] Jogo TETRIS - feito em C
Por
MIC_DUARTE, em C/C++
Este tópico foi arquivado e está fechado para novas respostas.
Por
MIC_DUARTE, em C/C++
Ao usar o fórum, você concorda com nossos Termos e condições.