Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
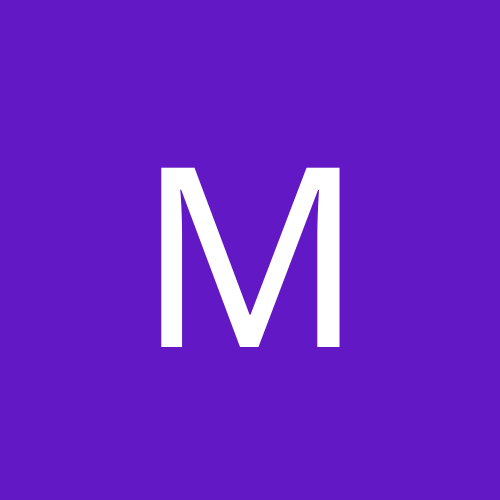
[Resolvido] ORM Doctrine 2
Por
Marcelo_Delta, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
Marcelo_Delta, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.