Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
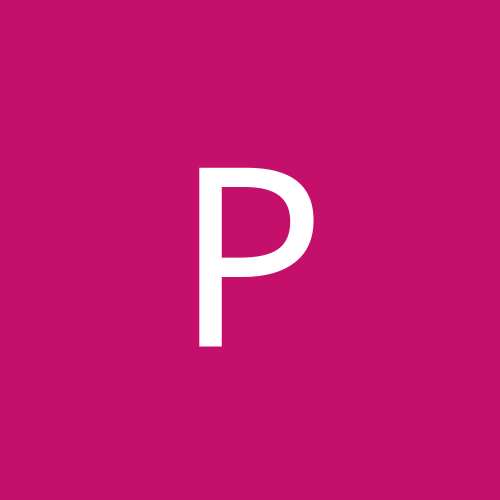
[Resolvido] Encriptção
Por
Pedroalves, em .NET
Este tópico foi arquivado e está fechado para novas respostas.
Por
Pedroalves, em .NET
Ao usar o fórum, você concorda com nossos Termos e condições.