Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
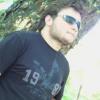
Persistir em Banco de Dados
Por
João Marcos Ferlini, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
João Marcos Ferlini, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.