Arquivado
Este tópico foi arquivado e está fechado para novas respostas.
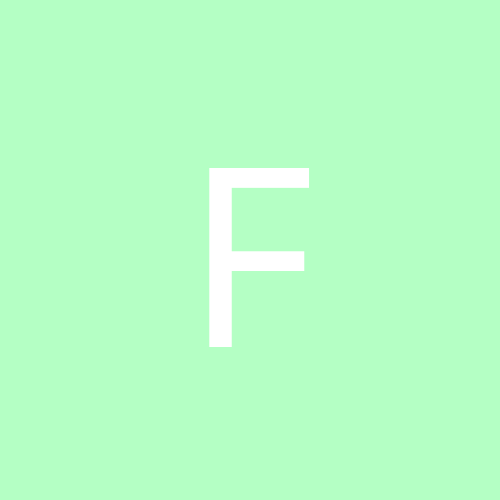
Sistema
Por
fsales_123, em PHP
Este tópico foi arquivado e está fechado para novas respostas.
Por
fsales_123, em PHP
Ao usar o fórum, você concorda com nossos Termos e condições.